Your team just rolled out an exciting new feature after months of development. The launch goes live, but almost immediately, users start reporting issues. The login page is broken. Profiles aren’t loading. And the payment system that worked yesterday? It’s failing across the board.
This is every development team’s nightmare. Even small code changes can break previously working functionality, leading to frustrated users, lost revenue, and damage to your brand’s reputation. In fact, bugs found in production cost 5x more to fix than those caught earlier.
These “regression bugs” not only frustrate users but can severely damage trust in your product and brand.
This is exactly what you need regression testing for.
So, what is regression testing? It’s a software testing practice that ensures recent code changes haven’t introduced new bugs into existing functionality.
In this guide, we’ll cover everything you need to know about regression testing—what it is, why it’s important, and how to implement it effectively in modern software development.
What is Regression Testing
Regression testing is a type of software testing that verifies that code changes haven’t adversely affected existing functionality. The term “regression” refers to the potential for software to regress—or revert—to a buggy state after modifications have been made to the codebase.
In software development, even a minor update can unintentionally affect unrelated parts of the application. Regression testing identifies these side effects early, ensuring stability and preventing new defects from being introduced into previously tested code.
Many sometimes confuse regression testing and retesting. It’s important to distinguish between the two:
- Regression testing: Ensures changes haven’t broken previously working features
- Retesting: Specifically verifies that a reported bug has been fixed
While they may seem similar, regression testing casts a much wider net, looking for unexpected issues across the application, while retesting targets a specific, known issue.
Example
Imagine you own an online store.
- Last week, a customer reported that the “Apply Coupon” button wasn’t working. Your dev team fixed it.
Retesting is when your QA team checks that this specific issue (the coupon button) now works as expected. - But while fixing that, there’s a chance the change accidentally broke something else, like the checkout page or the shopping cart total. Regression testing checks the rest of the site to make sure everything that used to work still works after the fix.
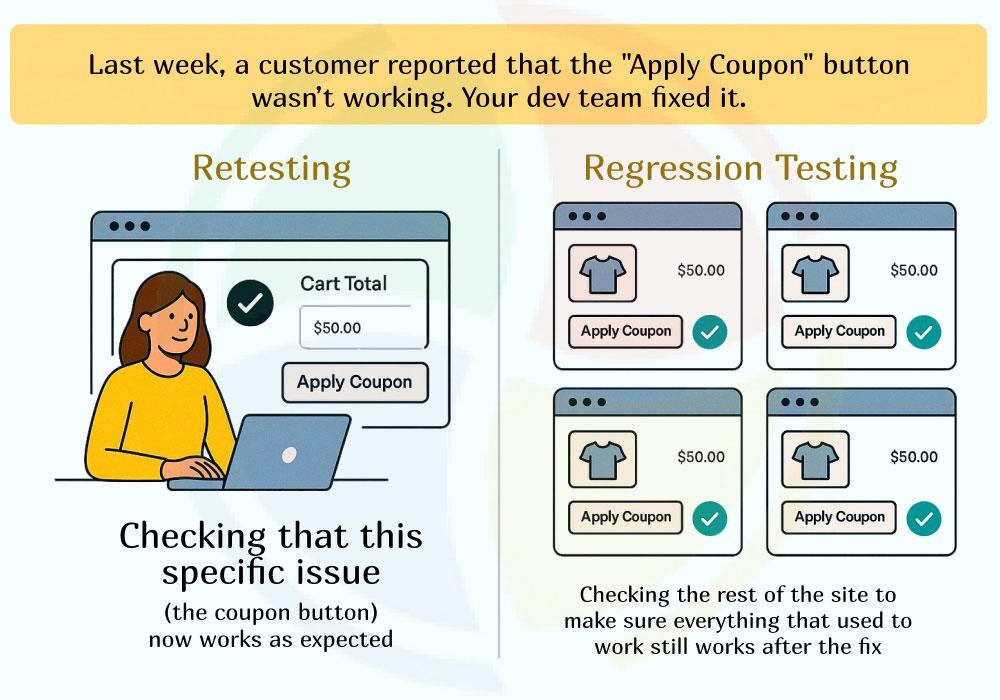
Why is Regression Testing Important?
In fast-paced Agile and DevOps environments, regression testing enables teams to release confidently, without sacrificing quality or user trust. In modern DevOps workflows, it especially helps in:
-> Continuous Integration/Deployment (CI/CD)
With multiple deployments a day, automated regression tests act as quality gates, ensuring stability while maintaining speed.
-> Microservice Architectures
In distributed systems, changes to one service can cause issues in others. Regression testing catches these inter-service bugs early.
-> Infrastructure as Code (IaC)
As infrastructure evolves, regression tests help confirm that applications behave consistently across different configurations.
-> High Release Velocity
Fast feature delivery demands confidence. Regression testing ensures updates don’t compromise system stability or add tech debt.
-> Feature Flags & Canary Releases
Verifies that new features work both when toggled on or off, and ensures partial rollouts don’t introduce unexpected behavior.
-> Shift-Left Testing
Enables developers to test earlier in the pipeline, validating code changes before submission and preventing bugs downstream.
-> Immediate Feedback
Automated regression tests give quick visibility into issues, supporting fast feedback loops and enabling rapid iteration.
When Should You Perform Regression Testing?

Regression testing should be an ongoing part of your development process as a checkpoint to ensure stability as your product evolves. It’s crucial during key moments such as:
-> After a new feature development
New code can unintentionally interfere with existing functionality—regression testing ensures nothing breaks as features are added.
-> Post bug fixes or patch updates
Fixing one issue can create another. Regression tests confirm the fix didn’t introduce new bugs elsewhere.
-> After code refactoring
Even when behavior shouldn’t change, restructuring code can cause hidden issues. Regression testing validates that everything still works as expected.
-> During each sprint or release cycle
Frequent releases mean frequent risk. Regular regression testing catches issues early without slowing down delivery.
-> After integrating third-party systems or APIs
External dependencies can behave unpredictably. Regression tests ensure your app remains stable after integration changes.
Regression Testing Techniques
Different scenarios call for different approaches to regression testing. Here are the main techniques used in the industry:
Unit Regression Testing
Unit regression testing focuses on the smallest testable parts of an application, typically individual functions or methods. When changes are made to a unit of code, regression tests verify that the unit still works correctly and that its interactions with other units haven’t been compromised.
When to Use | Benefits | Limitations |
• Minor code changes isolated to a single function or class • Dependencies are mocked or not impacted | • Fast and low-cost • Ideal for early-stage defect detection • Works well in TDD setups | • Doesn’t catch issues from interactions with other modules |
Partial Regression Testing
Partial regression testing (also called regional regression testing) is performed when changes are limited to specific modules or components. This approach tests both the modified component and any components that interact with it.
When to Use | Benefits | Limitations |
• Feature enhancements or bug fixes affecting multiple related components • During Agile sprints or module-level changes | • Efficient balance of coverage and speed • Reduces test execution time • Avoids redundant full-suite runs | • Risk of missing bugs in unrelated but indirectly affected areas |
Complete Regression Testing
Complete regression testing involves retesting the entire application, regardless of where changes were made. This comprehensive approach provides maximum coverage but requires significant time and resources.
When to Use | Benefits | Limitations |
• Major releases or codebase overhauls • After large-scale refactoring or code merges • In safety-critical applications | • Maximum confidence in software stability • Detects hard-to-spot side effects • Comprehensive | • Time-consuming • High resource usage • Not scalable without automation |
Regression Testing Process: A Step-by-Step Guide
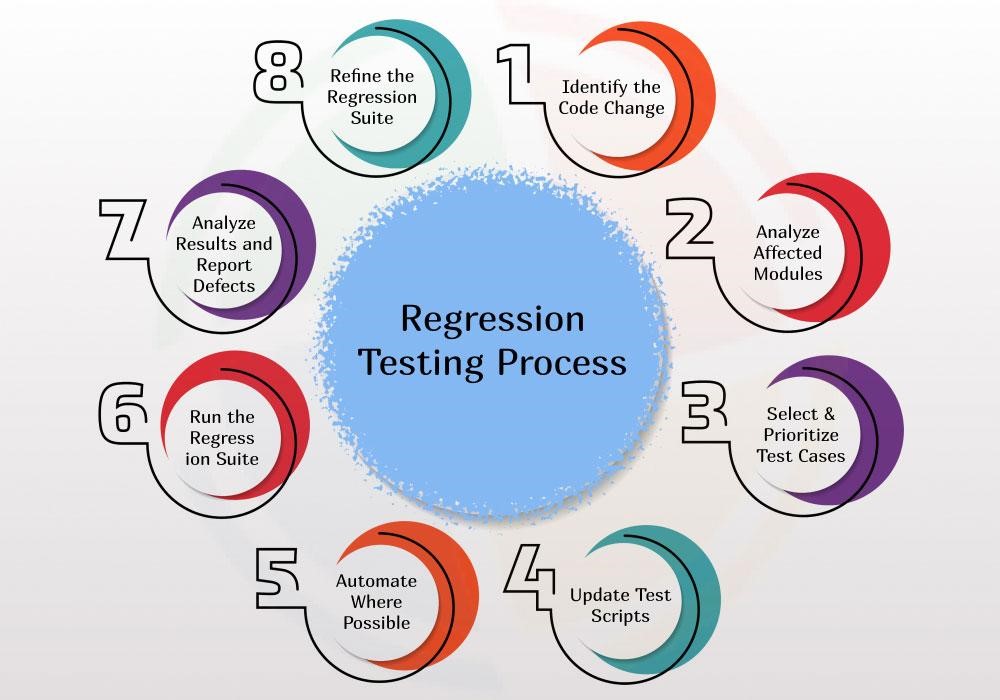
Here’s how to implement an effective, scalable regression testing strategy—step by step.
Step 1: Identify the Code Change
Begin by documenting all code changes, including new features, bug fixes, refactoring, or updates to libraries and dependencies. Use version control tools (like Git) to identify what exactly changed and why.
Understand the purpose and scope of each modification. This helps testers align their strategy with development priorities and ensures no risky area goes untested.
Step 2: Analyze Affected Modules
Once changes are identified, evaluate both direct and indirect impacts. Start with the modified modules, then trace dependencies—such as shared libraries, API endpoints, or UI components—that might be influenced.
Assess the risk level of each potentially affected area, especially those touching databases, user interfaces, or third-party integrations. Conducting a risk assessment informs the breadth and depth of your testing.
Step 3: Select & Prioritize Test Cases
Choose test cases that validate the updated functionality and its related dependencies. Prioritize these based on business impact, frequency of use, and historical defect data.
Focus on critical workflows and user journeys where bugs would be most costly. Also include tests for previously problematic components to catch recurring regressions.
Step 4: Update Test Scripts
Before execution, review and revise test cases to reflect the current state of the application. Update assertions and expected outcomes to align with legitimate changes.
Add new tests to cover added functionality and remove obsolete tests tied to deprecated features. Ensure test data is current, realistic, and relevant to production-like scenarios.
Step 5: Automate Where Possible
Manual regression testing quickly becomes unsustainable in fast-moving environments. Identify stable, repeatable test cases for automation, particularly for smoke tests, critical paths, and high-volume scenarios.
Use frameworks and regression testing tools like Selenium, Cypress, or JUnit, and integrate your automated suites into CI/CD pipelines to enable fast, consistent feedback. Be mindful of ROI, automate where it saves time, not just for the sake of it.
Step 6: Run the Regression Suite
Execute your regression suite in environments that closely mimic production. Use standardized test data to ensure consistency across runs. Organize test execution to follow logical sequences, especially for end-to-end flows.
Document outcomes clearly and track test execution metrics like duration and pass rates. This visibility helps identify bottlenecks and performance regressions.
Step 7: Analyze Results and Report Defects
After test execution, compare actual results to expected outcomes. Log any deviations as defects with detailed context, including screenshots, logs, and reproduction steps.
Classify issues by severity and impact, regression bugs should be tracked distinctly from new feature bugs to spot trends.
Step 8: Refine the Regression Suite
Regression testing isn’t a “set it and forget it” process. After each cycle, improve your suite by adding tests that catch new types of bugs, removing redundant ones, and reordering tests for faster execution. Document your reasoning for all changes so future teams understand the logic behind the suite’s evolution.
Defining Effective Regression Test Cases
Not every test case belongs in a regression suite. Only those that ensure stability across critical and high-risk areas. Here’s how to define them effectively:
• Scenario-Based Test Design
Start by designing tests around real-world user scenarios. These simulate how users interact with the application and help ensure functionality remains consistent after code changes.
Rather than isolated unit tests, these scenarios focus on full workflows, such as “user login and purchase flow” or “form submission and backend processing.” They surface bugs that arise from component interactions, not just individual failures.
• Focus on High-Use and High-Risk Features
Prioritize areas that are both frequently used and business-critical. For example, your regression suite should always include payment processing, user registration, or data entry modules.
Additionally, any module with a history of bugs or complex logic deserves focused regression coverage. This helps reduce the likelihood of high-impact defects reaching production.
• Maintain and Refactor Over Time
Regression suites can quickly become bloated or outdated. Regularly audit test cases to ensure they’re still relevant and valuable. Remove obsolete tests tied to deprecated features, refactor flaky ones, and consolidate redundant tests.
As the application evolves, so should your test cases. Keeping them lean, targeted, and efficient ensures long-term test health and better ROI on your QA investment.
Common Challenges in Regression Testing
While regression testing is essential, it comes with several challenges that can impact speed and accuracy:
-> Time and Resource Constraints
As applications scale, manual regression becomes slow and resource-heavy, delaying releases without automation.
-> Test Maintenance and Flaky Tests
Frequent code changes can break test scripts or cause inconsistent results, requiring ongoing maintenance to keep automation reliable.
-> Complex Dependencies
Third-party APIs and services can introduce regressions that are hard to trace, making comprehensive testing more difficult.
-> Test Suite Bloat
Over time, test suites can become overloaded with outdated or low-value tests, leading to longer execution times and reduced efficiency.
Benefits and Drawbacks of Regression Testing
Aspect | Benefits | Drawbacks |
Product Quality | Ensures software stability by catching bugs early | — |
Defect Detection | Identifies issues before production to reduce costly fixes | — |
Agile & CI/CD Support | Enables faster, reliable releases through automation | — |
Confidence | Builds trust for faster innovation and deployment | — |
Resource Use | — | Manual testing is time- and labor-intensive |
Maintenance | — | Automated tests need regular updates, requiring ongoing effort |
Test Suite Size | — | Test suites can become bloated, slowing execution and feedback cycles |
Setup Complexity | — | Automation frameworks and CI/CD integrations require upfront investment |
How to Scale Regression Testing Effectively
As your codebase and test coverage grow, so does the complexity of regression testing. To keep it efficient and reliable at scale:
-> Avoid Test Suite Bloat
Regularly audit and remove redundant, obsolete, or low-value test cases to streamline execution without losing coverage.
-> Adopt Parallel Test Execution
Speed up regression cycles by running tests in parallel across multiple environments or containers, especially useful in CI/CD pipelines.
-> Leverage Cloud Testing Infrastructure
Cloud-based platforms allow scalable execution across various devices, browsers, and configurations, reducing infrastructure overhead.
-> Use Containerization for Consistency
Running tests in containers (e.g., Docker) ensures consistent environments, minimizes flaky results, and improves portability.
-> Prioritize Test Cases
Use risk-based testing strategies to run high-impact or business-critical test cases first, optimizing feedback without running the entire suite every time.
-> Integrate Smart Test Selection
Modern tools can detect impacted areas of code and run only relevant regression tests, reducing execution time while maintaining accuracy.
Test Automation in Regression Testing: Why it Matters
As your software grows complex and the releases become more frequent, manual regression testing quickly becomes impractical. In short, they aren’t as sustainable. Which means you have only one answer–automation.
Automation testing allows you to execute large test suites rapidly and repeatedly, catching regressions early in development cycles. They are especially valuable for repetitive, high-volume scenarios like smoke tests, sanity checks, and critical user flows. They reduce human error and free testers to focus on exploratory testing and complex scenarios that require human judgment.
For teams aiming to deliver high-quality software at speed, investing in automation testing services is a competitive necessity today.
Instead of spending hours (or days) verifying existing functionality by hand, automation allows for:
- Faster feedback loops: Developers get near-instant insights into whether recent changes introduced any regressions.
- Scalability: Test coverage can grow with your codebase without increasing manual effort.
- Cost efficiency: While automation has an upfront cost, it reduces the long-term expense of repeated manual testing.
- Reliability: Automated tests eliminate human error, ensuring consistent validation of features across environments.
Expert implementation matters, though. Many teams struggle with building maintainable automation frameworks or find themselves dealing with flaky tests that create more problems than they solve.
Working with experienced automation testers can help you avoid these common pitfalls and build regression suites that deliver reliable results with minimal maintenance overhead.
How Aegis Helps with Scalable Regression Testing
Regression testing is a cornerstone of high-quality software delivery, but doing it manually isn’t sustainable, especially as your application scales. A structured, intelligent approach powered by test automation is essential in modern Agile and DevOps environments. This means identifying high-risk areas, selecting the right test cases, and seamlessly integrating automated tests within CI/CD workflows.
At Aegis, we help organizations scale their regression testing efforts through customized test automation services designed to fit seamlessly into fast-paced CI/CD pipelines.
Using tools like Selenium, Cypress, and cloud-native test environments, we create scalable, low-maintenance solutions that validate every release, fast and reliably. We prioritize high-impact test cases to optimize test execution time, reduce suite bloat, and catch regressions early.
Through cloud-based infrastructure, parallel execution, and containerized environments, we enable scalable, reliable test runs across diverse devices and configurations. With proactive maintenance and continuous refinement, our automation testing services ensure your test suites evolve with your application, minimizing flakiness and maximizing ROI.
Ready to streamline your regression testing with proven automation strategies?
Talk to our QA specialists to understand how automating regression testing can help you achieve the right balance of coverage, speed, and reliability for your specific development needs.
FAQs
What is QA regression testing?
QA regression testing is the process of verifying that recent code changes have not negatively impacted existing software functionality, ensuring consistent quality.
What is the STLC life cycle?
The Software Testing Life Cycle (STLC) defines the phases involved in testing a software application, from requirement analysis to test closure.
Is regression testing manual or automated?
Regression testing can be performed both manually and through automation testing services. However, test automation is often preferred for faster, repeatable, and scalable regression cycles.
What is bug life cycle?
The bug life cycle describes the stages a defect goes through—from discovery and reporting to fixing, retesting, and closure.
What is a real-life example of regression?
In a B2B SaaS platform, updating the user permissions module to add new roles might accidentally disrupt existing report access controls. Regression testing ensures these core permissions continue to work smoothly after the change.