Achieving rapid, reliable delivery of Node.js applications requires mastering continuous integration and delivery.
This comprehensive guide explores streamlining Azure DevOps services pipelines for Node.js projects using proven automation techniques, cutting-edge tools, and battle-tested best practices. Follow along to transform deployment dread into frictionless flows.
Overview of DevOps and CI/CD
First, what exactly is CI/CD and how does it fit into DevOps culture? DevOps emphasizes cross-functional collaboration, lean processes, and automation to improve software delivery speed and reliability.
CI/CD specifically brings automation to the build, test, and deployment process through orchestrated pipelines. This takes manual, failure-prone deployment steps and shifts them earlier into a standardized workflow.
The “continuous” aspects refer to constantly maintaining quality through automated validation at each stage, ongoing monitoring, and incremental improvements. The end goal is minimizing risk and maximizing velocity.
For Node.js apps, CI/CD is invaluable for catching regressions faster, reducing deployment headaches, and enabling rapid iteration. Let’s explore the key elements for building world-class pipelines.
Benefits of CI/CD Pipelines
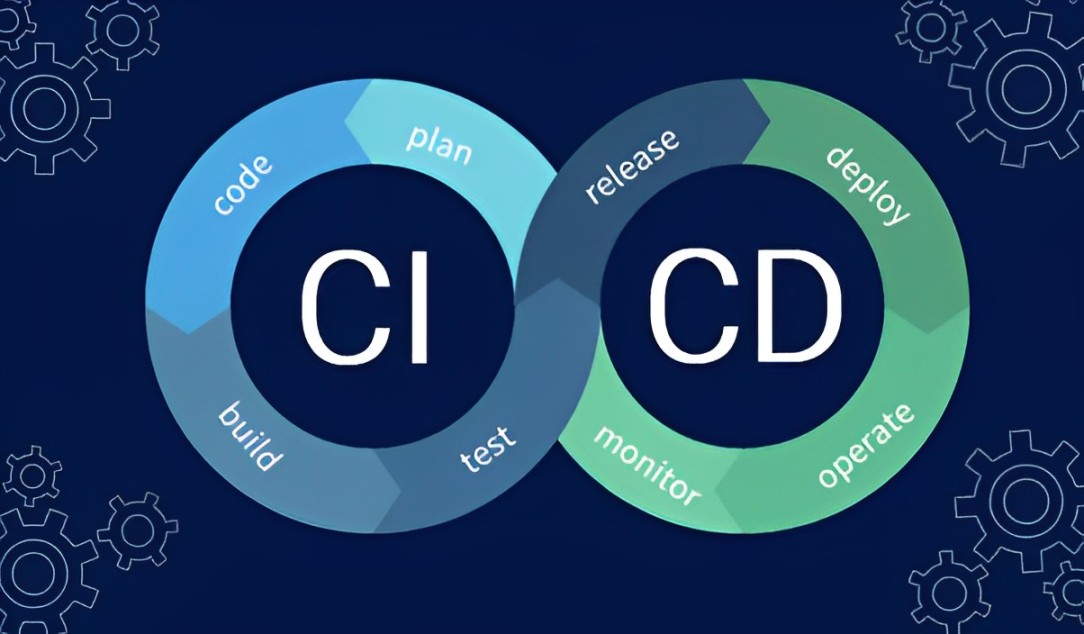
Implementing continuous integration and continuous delivery pipelines brings many key benefits:
CI/CD automation significantly reduces the manual workload involved in deploying applications repeatedly.
All the steps of building, testing, provisioning infrastructure, and promoting code get handled automatically based on triggers and policies. This frees up developer time and energy dramatically.
The quick feedback loops built into pipelines through extensive automated testing catch bugs and regressions much earlier in the process.
Issues can be fixed immediately before additional layers of code are built on top. This prevents small problems from accumulating into major headaches down the line.
CI/CD standardizes and codifies both application configuration and infrastructure provisioning for vastly improved consistency across environments.
There are no more snowflake production servers that have drifted. Each stage closely matches the production setup.
Pipelines provide easy rollback capabilities should any promotions fail. New versions can be automatically retracted, and the last known good build redeployed quickly. This reduces the pain and risk associated with a bad deployment.
The immutable artifact trails generated by CI/CD systems facilitate complete audibility and lineage tracking.
There is never a question about what changed when and why thanks to pipeline tooling. This satisfies compliance requirements.
By shifting testing and security scanning earlier into pipelines, risks are identified proactively rather than right before production deployment.
Issues can be fixed incrementally rather than derailing at the finish line.
Lastly, implementing small changes frequently reduces risk rather than massive, batched updates.
Code is constantly production-ready thanks to the batteries of tests enabling continuous delivery.
Core Components of a CI/CD Pipeline
While implementations may differ significantly, mature CI/CD pipelines generally share some common core components:
Source Control
All source code, configurations, scripts, and other artefacts are checked into a version control system like Git. This provides immutable versioning, change tracking, collaboration features like code reviews, and branching strategies.
Build Automation
The process of compiling code, transpiring languages, bundling dependencies, generating final deployment artefacts, and more is entirely automated consistently.
Testing
Extensive automated test suites validate changes at multiple levels like unit, integration, performance, security, etc. before further promotion. Testing provides safety nets at each pipeline stage.
Deployment Automation
Code and infrastructure changes flow through staging environments into production automatically through promotion policies, with no manual intervention.
Infrastructure as Code
All backend infrastructure and configurations for servers, databases, networks, etc. are defined in files and provisioned on-demand—no more configuration drift.
Monitoring
End-to-end telemetry from code to production provides pipeline visibility. Logging, metrics, and tracing identify issues and optimization opportunities.
Collaboration
Cross-functional teams collaborate closely across the full application lifecycle from development to operations using shared tools and practices.
These core disciplines reinforce each other to enable continuous delivery flows. However, meaningful implementation requires integrating the latest tools and architectures.
Source Control Management with Git
Robust source control management provides the foundation for reliable CI/CD pipelines:
Git has become the ubiquitous standard version control system due to its distributed architecture, branching capabilities, access controls, and strong community support.
All artefacts required to build, deploy and run applications – source code, configurations, scripts, docs – are checked into Git repositories for tracking.
Git facilitates collaboration through features like access controls, code reviews, issue tracking, and wikis to coordinate teams.
Webhooks can automatically trigger actions like CI pipeline jobs whenever changes are pushed to certain branches. This enables automation based on code activity.
Tags, semantic versioning and commit messages provide context around changes and releases to streamline management at scale.
With consistent Git processes, pipelines extract and act on the right versions of all needed artefacts in an automated fashion. Git provides a unified single source of truth.
Automated Building and Testing
Automating the build, test, and release processes is crucial for maintaining quality and speed as application scale and team sizes grow.
For Node.js applications specifically, the build stage may handle steps like:
- Linting and formatting code for consistency
- Transpiling TypeScript down to vanilla JavaScript
- Bundling modules and assets using Webpack
- Generating final deployment artefacts like containers
Automated testing expands validation across multiple dimensions including:
- Unit testing with frameworks like Mocha, and Jest to validate functions and classes
- Integration testing frameworks like SuperTest for verifying APIs and services
- Functional testing UI flows with Selenium, Cypress, Playwright
- Load and performance testing with k6, Artillery
- Dynamic and static analysis security scanning with tools like npm audit
By shifting these critical activities earlier into automated pipelines rather than right before deployment, issues can be caught continuously. Extensive testing provides safety nets at each stage of pipeline workflows.
Continuous Integration (CI)
The practice of continuous integration addresses a major source of problems – long-lived branches:
Integrating work frequently through small changes sets catches regressions and conflicts rapidly as code progresses rather than further down the line.
Automating build and test workflows on every commit or merge request provides quick feedback on change quality before merging.
Maintaining a healthy master or mainline branch also ensures the shared baseline is always releasable.
For Node.js applications, continuous integration is invaluable for identifying issues early through extensive automated testing anytime code changes.
The key is continually integrating developer work into the mainline via pull requests coupled with batteries of automated checks pre-merge. Done right, continuous integration powerfully complements continuous delivery.
Automated Deployment Tools
Specializing tools have emerged to provide automation orchestrating code through the progression of environments to production:
Powerful features include templating infrastructure, modeling deployments through code, facilitating rapid rollbacks, managing secrets and access controls, provisioning resources on-demand, and more.
For Node.js applications, options like Jenkins, CircleCI, TravisCI, GitHub Actions, and many others help automate promotion flows into testing, staging, and production environments.
These deployment automation tools also integrate cleanly with infrastructure as code solutions to manage backend cloud provisioning and immutability.
Combined they provide a flexible, configurable framework for promoting applications from commit to customer automatically under defined conditions and approvals.
Continuous Delivery and Continuous Deployment
Two similar but distinct patterns emerge in mature CI/CD pipelines:
Continuous Delivery means developers produce production-ready builds continuously that can be released to customers manually.
Continuous Deployment goes further by fully automating the release process into production environments based on events like passing tests.
In practice, most teams land somewhere in the middle – utilizing extensive test automation to keep code releasable but requiring final approvals before full production deployment.
For Node.js applications, implementing CI/CD allows balancing rapid innovation with governable, low-risk delivery tuned precisely to organizational needs.
Infrastructure as Code & Configuration Management
Manually configuring and maintaining servers, databases, networks, and other infrastructure is a recipe for reliability disasters:
Infrastructure as code techniques help address this by managing infrastructure needs in versioned files using declarative definitions – HCL, YAML, JSON rather than UIs.
Treating configurations as code allows repeating environments reliably through automated provisioning tools like Terraform, CloudFormation, and Pulumi.
The huge benefit is no more production of snowflake servers! Infrastructure variation is codified and managed through pipelines rather than manual changes.
For Node.js applications, defining and evolving infrastructure as files checked into Git is essential for maintaining integrity across environments throughout continuous delivery workflows.
Monitoring and Observability
End-to-end pipeline visibility is critical for understanding failures and identifying optimization opportunities:
Centralized structured logging provides insights into all steps and failures during executions.
Performance monitoring illuminates lead times through various pipeline stages – build, test, release, etc.
Granular metrics help locate bottlenecks like slow test suites limiting release velocity.
Distributed tracing maps deployments to infrastructure changes and application response.
Proactive alerting on regressions or incidents enables rapid mitigation.
Monitoring provides the feedback needed to improve continually.
Securing CI/CD Pipelines
With pipelines wielding immense power over infrastructure, security is mandatory:
Strict least-privilege controls must be enforced on all user roles and service accounts. Limit blast radius from compromise.
Secrets like API tokens should be securely stored in vaults with minimal access rather than exposed in plain text.
Automated security and license scanning at pipeline checkpoints help catch issues proactively.
Production infrastructure should be hardened by security groups, private networks/endpoints, VPNs, etc.
All pipeline activity should be monitored for anomalies indicating potential malicious use.
Short-lived ephemeral credentials help limit damage if leaked.
Holistic security measures are required to prevent pipeline compromise which could be catastrophic given their reach. Risk increases exponentially with velocity, so security cannot be an afterthought in CI/CD systems.
CI/CD Tooling for Node.js Apps
CI/CD tooling tests code changes builds and packages apps, and ships updates quickly and reliably.
For CI, tools like Jenkins, CircleCI, TravisCI, and GitHub Actions run automated test suites every time new code is committed to source control.
Unit, integration, load, and other tests run to validate the changes on the CI server. If any test fails, the commit is rejected to prevent bugs. This provides a safety net ensuring all code merged meets quality standards.
On the CD side, the same tools can automate release workflows. The CI process produces a verified app package that can trigger deployment.
Using infrastructure-as-code tools like Terraform or CloudFormation, the CD pipeline provisions infrastructure on Kubernetes or VMs.
It deploys the Node app package onto servers and performs automated smoke testing to validate the environments. If any stage fails, the pipeline rolls back.
Hire nodejs Developers can configure CI/CD workflows through the tool’s interface or as code in YAML files checked into repositories.
Webhooks connect GitHub commits to triggering pipelines. Logs and notifications keep teams updated on deployments.
Leveraging CI/CD automation provides immense advantages. Code is rigorously tested before reaching production. Deployments are consistent and low risk. Issues are caught early. New features ship faster.
Engineers avoid manual toil updating servers. CI/CD lets developers focus on writing code rather than ops. For smooth DevOps with Node.js, implementing continuous pipelines is essential.
This comprehensive guide explored streamlining modern CI/CD pipelines for Node.js apps using proven techniques like test automation, infrastructure as code, and version control.
Approached holistically, each discipline complements the next to form an efficient software delivery machine. Developers gain the flexibility to sustainably deliver innovations safely.
Of course, realities like technical debt, legacy systems, and change resistance can slow progress. However, incremental improvement towards mature CI/CD ultimately enables Node.js teams to ship faster without compromise or chaos.
How have you evolved deployment practices for Node.js apps? What lessons or tools have been most impactful? Please share your experiences below!